Adminer - Extensions
Both Adminer and Editor offers support for extensions. It is possible to overwrite some default Adminer functionality by a custom code. All you need is to define the adminer_object
function which returns an object extending the basic Adminer
class. Then just include the original adminer.php
or editor.php
:
<?php
function adminer_object() {
class AdminerSoftware extends Adminer {
function name() {
// custom name in title and heading
return 'Software';
}
function permanentLogin() {
// key used for permanent login
return 'aed8555186fb6ea2b6ca793c7cb05590';
}
function credentials() {
// server, username and password for connecting to database
return array('localhost', 'ODBC', '');
}
function database() {
// database name, will be escaped by Adminer
return 'software';
}
function login($login, $password) {
// validate user submitted credentials
return ($login == 'admin' && $password == 'oySrN9Jv');
}
function tableName($tableStatus) {
// tables without comments would return empty string and will be ignored by Adminer
return h($tableStatus['Comment']);
}
function fieldName($field, $order = 0) {
// only columns with comments will be displayed and only the first five in select
return ($order <= 5 && !preg_match('~_(md5|sha1)$~', $field['field']) ? h($field['comment']) : '');
}
}
return new AdminerSoftware;
}
include './editor.php';
To create an Adminer Editor customization for other drivers than MySQL, you have to define own loginForm
method and fill the auth[driver]
field value by your driver (pgsql
, sqlite
, ...).
You usually also need to implement the database
method.
Example: editor/sqlite.php.
Adminer also supports plugins which are ready to use extensions.
API reference
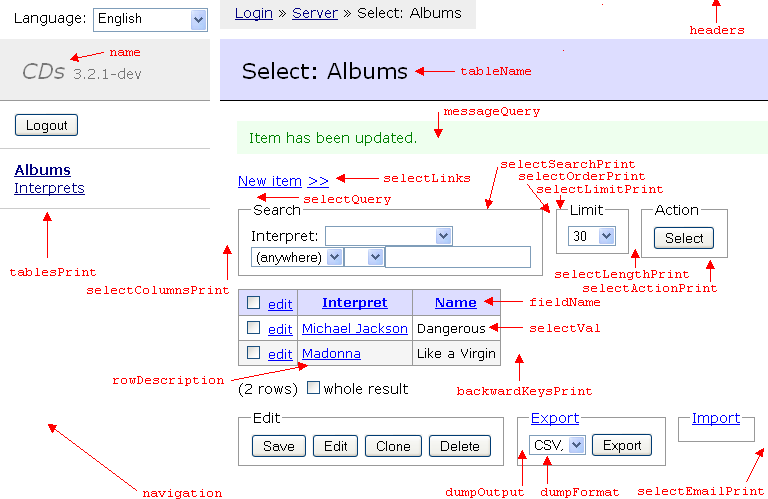
The object can overwrite following methods:
- string Adminer::name() - Name in title and navigation
- array Adminer::credentials() - Connection parameters
- array Adminer::connectSsl() - Get SSL connection options
- string Adminer::permanentLogin(bool $create) - Get key used for permanent login
- string Adminer::bruteForceKey() - Return key used to group brute force attacks; behind a reverse proxy, you want to return the last part of X-Forwarded-For
- string Adminer::serverName(string $server) - Get server name displayed in breadcrumbs
- string Adminer::database() - Identifier of selected database
- array Adminer::databases(bool $flush) - Get cached list of databases
- array Adminer::schemas() - Get list of schemas
- float Adminer::queryTimeout() - Specify limit for waiting on some slow queries like DB list
- null Adminer::headers() - Headers to send before HTML output
- array Adminer::csp() - Get Content Security Policy headers
- bool Adminer::head() - Print HTML code inside <head>
- array Adminer::css() - Get URLs of the CSS files
- null Adminer::loginForm() - Print login form
- string Adminer::loginFormField(string $name, string $heading, string $value) - Get login form field
- mixed Adminer::login(string $login, string $password) - Authorize the user
- string Adminer::tableName(array $tableStatus) - Table caption used in navigation and headings
- string Adminer::fieldName(array $field, int $order) - Field caption used in select and edit
- null Adminer::selectLinks(array $tableStatus, string $set) - Print links after select heading
- array Adminer::foreignKeys(string $table) - Get foreign keys for table
- array Adminer::backwardKeys(string $table, string $tableName) - Find backward keys for table
- null Adminer::backwardKeysPrint(array $backwardKeys, array $row) - Print backward keys for row
- string Adminer::selectQuery(string $query, float $start, bool $failed) - Query printed in select before execution
- string Adminer::sqlCommandQuery(string $query) - Query printed in SQL command before execution
- string Adminer::rowDescription(string $table) - Description of a row in a table
- array Adminer::rowDescriptions(array $rows, array $foreignKeys) - Get descriptions of selected data
- string Adminer::selectLink(string $val, array $field) - Get a link to use in select table
- string Adminer::selectVal(string $val, string $link, array $field, array $original) - Value printed in select table
- string Adminer::editVal(string $val, array $field) - Value conversion used in select and edit
- null Adminer::tableStructurePrint(array $fields) - Print table structure in tabular format
- null Adminer::tableIndexesPrint(array $indexes) - Print list of indexes on table in tabular format
- null Adminer::selectColumnsPrint(array $select, array $columns) - Print columns box in select
- null Adminer::selectSearchPrint(array $where, array $columns, array $indexes) - Print search box in select
- null Adminer::selectOrderPrint(array $order, array $columns, array $indexes) - Print order box in select
- null Adminer::selectLimitPrint(string $limit) - Print limit box in select
- null Adminer::selectLengthPrint(string $text_length) - Print text length box in select
- null Adminer::selectActionPrint(array $indexes) - Print action box in select
- bool Adminer::selectCommandPrint() - Print command box in select
- bool Adminer::selectImportPrint() - Print import box in select
- null Adminer::selectEmailPrint(array $emailFields, array $columns) - Print extra text in the end of a select form
- array Adminer::selectColumnsProcess(array $columns, array $indexes) - Process columns box in select
- array Adminer::selectSearchProcess(array $fields, array $indexes) - Process search box in select
- array Adminer::selectOrderProcess(array $fields, array $indexes) - Process order box in select
- string Adminer::selectLimitProcess() - Process limit box in select
- string Adminer::selectLengthProcess() - Process length box in select
- bool Adminer::selectEmailProcess(array $where, array $foreignKeys) - Process extras in select form
- string Adminer::selectQueryBuild(array $select, array $where, array $group, array $order, int $limit, int $page) - Build SQL query used in select
- string Adminer::messageQuery(string $query, string $time, bool $failed) - Query printed after execution in the message
- array Adminer::editFunctions(array $field) - Functions displayed in edit form
- string Adminer::editInput(string $table, array $field, string $attrs, string $value) - Get options to display edit field
- string Adminer::editHint(string $table, array $field, string $value) - Get hint for edit field
- string Adminer::processInput(array $field, string $value, string $function) - Process sent input
- array Adminer::dumpOutput() - Returns export output options
- array Adminer::dumpFormat() - Returns export format options
- null Adminer::dumpDatabase(string $db) - Export database structure
- null Adminer::dumpTable(string $table, string $style, int $is_view) - Export table structure
- null Adminer::dumpData(string $table, string $style, string $query) - Export table data
- string Adminer::dumpFilename(string $identifier) - Set export filename
- string Adminer::dumpHeaders(string $identifier, bool $multi_table) - Send headers for export
- string Adminer::importServerPath() - Set the path of the file for webserver load
- bool Adminer::homepage() - Print homepage
- null Adminer::navigation(string $missing) - Prints navigation after Adminer title
- null Adminer::databasesPrint(string $missing) - Prints databases list in menu
- null Adminer::tablesPrint(array $tables) - Prints table list in menu
It is possible to use following global functions.
- Min_DB connection() - Get database connection
- Adminer adminer() - Get Adminer object
- string version() - Get Adminer version
- string idf_unescape(string $idf) - Unescape database identifier
- string escape_string(string $val) - Escape string to use inside ''
- string number(string $val) - Remove non-digits from a string
- string number_type() - Get regular expression to match numeric types
- null remove_slashes(array $process, bool $filter) - Disable magic_quotes_gpc
- string bracket_escape(string $idf, bool $back) - Escape or unescape string to use inside form []
- bool min_version(string $version, string $maria_db, Min_DB $connection2) - Check if connection has at least the given version
- string charset(Min_DB $connection) - Get connection charset
- string script(string $source, string $trailing) - Return <script> element
- string script_src(string $url) - Return <script src> element
- string nonce() - Get a nonce="" attribute with CSP nonce
- string target_blank() - Get a target="_blank" attribute
- string h(string $string) - Escape for HTML
- string nl_br(string $string) - Convert \n to <br>
- string checkbox(string $name, string $value, bool $checked, string $label, string $onclick, string $class, string $labelled_by) - Generate HTML checkbox
- string optionlist(array $options, mixed $selected, bool $use_keys) - Generate list of HTML options
- string html_select(string $name, array $options, string $value, string $onchange, string $labelled_by) - Generate HTML radio list
- string select_input(string $attrs, array $options, string $value, string $onchange, string $placeholder) - Generate HTML <select> or <input> if $options are empty
- string confirm(string $message, string $selector) - Get onclick confirmation
- null print_fieldset(string $id, string $legend, bool $visible) - Print header for hidden fieldset (close by </div></fieldset>)
- string bold(bool $bold, string $class) - Return class='active' if $bold is true
- string odd(string $return) - Generate class for odd rows
- string js_escape(string $string) - Escape string for JavaScript apostrophes
- null json_row(string $key, string $val) - Print one row in JSON object
- bool ini_bool(string $ini) - Get INI boolean value
- bool sid() - Check if SID is neccessary
- null set_password(string $vendor, string $server, string $username, string $password) - Set password to session
- string get_password() - Get password from session
- string q(string $string) - Shortcut for $connection->quote($string)
- array get_vals(string $query, mixed $column) - Get list of values from database
- array get_key_vals(string $query, Min_DB $connection2, bool $set_keys) - Get keys from first column and values from second
- array get_rows(string $query, Min_DB $connection2, string $error) - Get all rows of result
- array unique_array(array $row, array $indexes) - Find unique identifier of a row
- string escape_key(string $key) - Escape column key used in where()
- string where(array $where, array $fields) - Create SQL condition from parsed query string
- string where_check(string $val, array $fields) - Create SQL condition from query string
- string where_link(int $i, string $column, string $value, string $operator) - Create query string where condition from value
- string convert_fields(array $columns, array $fields, array $select) - Get select clause for convertible fields
- bool cookie(string $name, string $value, int $lifetime) - Set cookie valid on current path
- null restart_session() - Restart stopped session
- null stop_session(bool $force) - Stop session if possible
- mixed &get_session(string $key) - Get session variable for current server
- mixed set_session(string $key, mixed $val) - Set session variable for current server
- string auth_url(string $vendor, string $server, string $username, string $db) - Get authenticated URL
- bool is_ajax() - Find whether it is an AJAX request
- null redirect(string $location, string $message) - Send Location header and exit
- bool query_redirect(string $query, string $location, string $message, bool $redirect, bool $execute, bool $failed, string $time) - Execute query and redirect if successful
- Min_Result queries(string $query) - Execute and remember query
- bool apply_queries(string $query, array $tables, callback $escape) - Apply command to all array items
- bool queries_redirect(string $location, string $message, bool $redirect) - Redirect by remembered queries
- string format_time(float $start) - Format elapsed time
- string remove_from_uri(string $param) - Remove parameter from query string
- string pagination(int $page, int $current) - Generate page number for pagination
- mixed get_file(string $key, bool $decompress) - Get file contents from $_FILES
- string upload_error(int $error) - Determine upload error
- string repeat_pattern(string $pattern, int $length) - Create repeat pattern for preg
- bool is_utf8(string $val) - Check whether the string is in UTF-8
- string shorten_utf8(string $string, int $length, string $suffix) - Shorten UTF-8 string
- string format_number(int $val) - Format decimal number
- string friendly_url(string $val) - Generate friendly URL
- bool hidden_fields(array $process, array $ignore) - Print hidden fields
- null hidden_fields_get() - Print hidden fields for GET forms
- array table_status1(string $table, bool $fast) - Get status of a single table and fall back to name on error
- array column_foreign_keys(string $table) - Find out foreign keys for each column
- null enum_input(string $type, string $attrs, array $field, mixed $value, string $empty) - Print enum input field
- null input(array $field, mixed $value, string $function) - Print edit input field
- string process_input(one $field) - Process edit input field
- array fields_from_edit() - Compute fields() from $_POST edit data
- null search_tables() - Print results of search in all tables
- string dump_headers(string $identifier, bool $multi_table) - Send headers for export
- null dump_csv(array $row) - Print CSV row
- string apply_sql_function(string $function, string $column) - Apply SQL function
- string get_temp_dir() - Get path of the temporary directory
- resource file_open_lock(string $filename) - Open and exclusively lock a file
- file_write_unlock(resource $fp, string $data) - Write and unlock a file
- string password_file(bool $create) - Read password from file adminer.key in temporary directory or create one
- string rand_string() - Get a random string
- string select_value(string $val, string $link, array $field, int $text_length) - Format value to use in select
- bool is_mail(string $email) - Check whether the string is e-mail address
- bool is_url(string $string) - Check whether the string is URL address
- bool is_shortable(array $field) - Check if field should be shortened
- string count_rows(string $table, array $where, bool $is_group, array $group) - Get query to compute number of found rows
- array slow_query(string $query) - Run query which can be killed by AJAX call after timing out
- string get_token() - Generate BREACH resistant CSRF token
- bool verify_token() - Verify if supplied CSRF token is valid
- string on_help(string $command, bool $side) - Return events to display help on mouse over
- null edit_form(string $TABLE, array $fields, mixed $row, bool $update) - Print edit data form
Language specific functions.
- string get_lang() - Get current language
- string lang(string $idf, int $number) - Translate string
Driver specific functions:
- bool Min_DB::connect(string $server, string $username, string $password) - Connect to server
- bool Min_DB::set_charset(string $charset) - Sets the client character set
- string Min_DB::quote(string $string) - Quote string to use in SQL
- bool Min_DB::select_db(string $database) - Select database
- mixed Min_DB::query(string $query, bool $unbuffered) - Send query
- bool Min_DB::multi_query(string $query) - Send query with more resultsets
- Min_Result Min_DB::store_result() - Get current resultset
- bool Min_DB::next_result() - Fetch next resultset
- string Min_DB::result(string $query, int $field) - Get single field from result
- Min_Result::__construct(resource $result) - Constructor
- array Min_Result::fetch_assoc() - Fetch next row as associative array
- array Min_Result::fetch_row() - Fetch next row as numbered array
- object Min_Result::fetch_field() - Fetch next field
- Min_Result::__destruct() - Free result set
- string idf_escape(string $idf) - Escape database identifier
- string table(string $idf) - Get escaped table name
- mixed connect() - Connect to the database
- array get_databases(bool $flush) - Get cached list of databases
- string limit(string $query, string $where, int $limit, int $offset, string $separator) - Formulate SQL query with limit
- string limit1(string $table, string $query, string $where, string $separator) - Formulate SQL modification query with limit 1
- string db_collation(string $db, array $collations) - Get database collation
- array engines() - Get supported engines
- string logged_user() - Get logged user
- array tables_list() - Get tables list
- array count_tables(array $databases) - Count tables in all databases
- array table_status(string $name, bool $fast) - Get table status
- bool is_view(array $table_status) - Find out whether the identifier is view
- bool fk_support(array $table_status) - Check if table supports foreign keys
- array fields(string $table) - Get information about fields
- array indexes(string $table, string $connection2) - Get table indexes
- array foreign_keys(string $table) - Get foreign keys in table
- array view(string $name) - Get view SELECT
- array collations() - Get sorted grouped list of collations
- bool information_schema(string $db) - Find out if database is information_schema
- string error() - Get escaped error message
- string create_database(string $db, string $collation) - Create database
- bool drop_databases(array $databases) - Drop databases
- bool rename_database(string $name, string $collation) - Rename database from DB
- string auto_increment() - Generate modifier for auto increment column
- bool alter_table(string $table, string $name, array $fields, array $foreign, string $comment, string $engine, string $collation, string $auto_increment, string $partitioning) - Run commands to create or alter table
- bool alter_indexes(string $table, array $alter) - Run commands to alter indexes
- bool truncate_tables(array $tables) - Run commands to truncate tables
- bool drop_views(array $views) - Drop views
- bool drop_tables(array $tables) - Drop tables
- bool move_tables(array $tables, array $views, string $target) - Move tables to other schema
- bool copy_tables(array $tables, array $views, string $target) - Copy tables to other schema
- array trigger(string $name) - Get information about trigger
- array triggers(string $table) - Get defined triggers
- array trigger_options() - Get trigger options
- array routine(string $name, string $type) - Get information about stored routine
- array routines() - Get list of routines
- array routine_languages() - Get list of available routine languages
- string routine_id(string $name, array $row) - Get routine signature
- string last_id() - Get last auto increment ID
- Min_Result explain(Min_DB $connection, string $query) - Explain select
- int found_rows(array $table_status, array $where) - Get approximate number of rows
- array types() - Get user defined types
- array schemas() - Get existing schemas
- string get_schema() - Get current schema
- bool set_schema(string $schema) - Set current schema
- string create_sql(string $table, bool $auto_increment, string $style) - Get SQL command to create table
- string truncate_sql(string $table) - Get SQL command to truncate table
- string use_sql(string $database) - Get SQL command to change database
- string trigger_sql(string $table) - Get SQL commands to create triggers
- array show_variables() - Get server variables
- array process_list() - Get process list
- array show_status() - Get status variables
- string convert_field(array $field) - Convert field in select and edit
- string unconvert_field(array $field, string $return) - Convert value in edit after applying functions back
- bool support(string $feature) - Check whether a feature is supported
Min_Driver:
- Min_SQL::__construct(Min_DB $connection) - Create object for performing database operations
- Min_Result Min_SQL::select(string $table, array $select, array $where, array $group, array $order) - Select data from table
- bool Min_SQL::delete(string $table, string $queryWhere, int $limit) - Delete data from table
- bool Min_SQL::update(string $table, array $set, string $queryWhere, int $limit, string $separator) - Update data in table
- bool Min_SQL::insert(string $table, array $set) - Insert data into table
- bool Min_SQL::begin() - Begin transaction
- bool Min_SQL::commit() - Commit transaction
- bool Min_SQL::rollback() - Rollback transaction
- string Min_SQL::slowQuery(string $query, int $timeout) - Return query with a timeout
- string Min_SQL::convertSearch(string $idf, array $val, array $field) - Convert column to be searchable
- string Min_SQL::value(string $val, array $field) - Convert value returned by database to actual value
- string Min_SQL::quoteBinary(string $s) - Quote binary string
- string Min_SQL::warnings() - Get warnings about the last command
- string Min_SQL::tableHelp(string $name) - Get help link for table
Adminer specific functions:
- array select(Min_Result $result, Min_DB $connection2, array $orgtables) - Print select result
- array referencable_primary(string $self) - Get referencable tables with single column primary key except self
- array adminer_settings() - Get settings stored in a cookie
- array adminer_setting(string $key) - Get setting stored in a cookie
- bool set_adminer_settings(array $settings) - Store settings to a cookie
- null textarea(string $name, string $value, int $rows, int $cols) - Print SQL <textarea> tag
- null edit_type(string $key, array $field, array $collations, array $foreign_keys) - Print table columns for type edit
- string process_length(string $length) - Filter length value including enums
- string process_type(array $field, string $collate) - Create SQL string from field type
- array process_field(array $field, array $type_field) - Create SQL string from field
- string default_value(array $field) - Get default value clause
- string type_class(string $type) - Get type class to use in CSS
- null edit_fields(array $fields, array $collations, string $type, array $foreign_keys) - Print table interior for fields editing
- bool process_fields(array $fields) - Move fields up and down or add field
- string normalize_enum(array $match) - Callback used in routine()
- bool grant(string $grant, array $privileges, string $columns, string $on) - Issue grant or revoke commands
- null drop_create(string $drop, string $create, string $drop_created, string $test, string $drop_test, string $location, string $message_drop, string $message_alter, string $message_create, string $old_name, string $new_name) - Drop old object and create a new one
- string create_trigger(string $on, array $row) - Generate SQL query for creating trigger
- string create_routine(string $routine, array $row) - Generate SQL query for creating routine
- string remove_definer(string $query) - Remove current user definer from SQL command
- string format_foreign_key(array $foreign_key) - Format foreign key to use in SQL query
- null tar_file(string $filename, TmpFile $tmp_file) - Add a file to TAR
- int ini_bytes(string $ini) - Get INI bytes value
- string doc_link(array $paths, string $text) - Create link to database documentation
- string ob_gzencode(string $string) - Wrap gzencode() for usage in ob_start()
- string db_size(string $db) - Compute size of database
- null set_utf8mb4(string $create) - Print SET NAMES if utf8mb4 might be needed
Editor specific functions:
- string email_header(string $header) - Encode e-mail header in UTF-8
- bool send_mail(string $email, string $subject, string $message, string $from, array $files) - Send e-mail in UTF-8
- bool like_bool(array $field) - Check whether the column looks like boolean